C Programming Gate Questions and Answers Pdf
GATE CSE 2024 SET-2
Q1: Consider an array X that contains n positive integers. A subarray of X is defined to be a sequence of array locations with consecutive indices.
The C code snippet given below has been written to compute the length of the longest subarray of X that contains at most two distinct integers. The code has two missing expressions labelled (P) and (Q).
int first=0, second=0, len1=0, len2=0, maxlen=0; for (int i=0; i < n; i++) { if (X[i] == first) { len2++; len1++; } else if (X[i] == second) { len2++; len1 = (P) ; second = first; } else { len2 = (Q) ; len1 = 1; second = first; } if (len2 > maxlen) { maxlen = len2; } first = X[i]; }
Which one of the following options gives the CORRECT missing expressions?
(Hint: At the end of the i-th iteration, the value of len1 is the length of the longest subarray ending with X[i] that contains all equal values, and len2 is the length of the longest subarray ending with X[i] that contains at most two distinct values.)
- A) (P) len1+1 (Q) len2+1
- B) (P) 1 (Q) len1+1
- C) (P) 1 (Q) len2+1
- D) (P) len2+1 (Q) len1+1
Q2: What is the output of the following C program?
#include <stdio.h> int main() { double a[2] = {20.0, 25.0}, *p, *q; p = a; q = p + 1; printf("%d,%d", (int)(q - p), (int)(*q - *p)); return 0; }
- A) 4,8
- B) 1,5
- C) 8,5
- D) 1,8
Q3: Consider the following C function definition.
int fX(char *a) { char *b = a; while (*b) b++; return b - a; }
- A) The function call fX(“abcd”) will always return a value
- B) Assuming a character array c is declared as char c[] = “abcd” in main(), the function call fX(c)will always return a value
- C) The code of the function will not compile
- D) Assuming a character pointer c is declared as char *c = “abcd” in main(), the function call fX(c)will always return a value
Q4: Consider the following C program. Assume parameters to a function are evaluated from right to left.
#include <stdio.h> int g(int p) { printf("%d", p); return p; } int h(int q) { printf("%d", q); return q; } void f(int x, int y) { g(x); h(y); } int main() { f(g(10), h(20)); }
- A) 20101020
- B) 10202010
- C) 20102010
- D) 10201020
GATE CSE 2024 SET-1
Q5: Consider the following C function definition.
int f(int x, int y) { for (int i = 0; i < y; i++) { x = x + x + y; } return x; }
- A) If the inputs are x=20, y=10, then the return value is greater than 220
- B) If the inputs are x=20, y=20, then the return value is greater than 220
- C) If the inputs are x=20, y=10, then the return value is less than 210
- D) If the inputs are x=10, y=20, then the return value is greater than 220
Q6: Consider the following C program:
#include <stdio.h> void fX(); int main() { fX(); return 0; } void fX() { char a; if ((a = getchar()) != '\n') fX(); if (a != '\n') putchar(a); }
Assume that the input to the program from the command line is 1234 followed by a newline character. Which one of the following statements is CORRECT?
- A) The program will not terminate
- B) The program will terminate with no outpu
- C) The program will terminate with 4321 as output
- D) The program will terminate with 1234 as output
Q7: Consider the following C program:
#include <stdio.h> int main() { int a = 6; int b = 0; while (a < 10) { a = a / 12 + 1; a += b; } printf("%d", a); return 0; }
Which one of the following statements is CORRECT?
- A) The program prints 9 as output
- B) The program prints 10 as output
- C) The program gets stuck in an infinite loop
- D) The program prints 6 as output
GATE CSE 2023
Q8: Consider the following program:
#include <stdio.h> int f1(); int f2(int X); int f3(); int main() { f1(); f2(2); f3(); return 0; } int f1() { return 1; } int f2(int X) { f3(); if (X == 1) return f1(); else return (X * f2(X - 1)); } int f3() { return 5; }
Which one of the following options represents the activation tree corresponding to the main function?
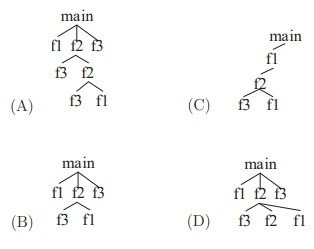
- A) A
- B) B
- C) C
- D) D
Q9 : The integer value printed by the ANSI-C program given below is __.
#include < stdio.h > int funcp(){ static int x = 1; x++; return x; } int main(){ int x,y; x = funcp(); y = funcp()+x; printf("%d\n", (x+y)); return 0; }
GATE CSE 2022
Q10: What is printed by the following ANSI C program?
#include <stdio.h> int main(int argc, char *argv[]) { char a = 'P'; char b = 'x'; char c = (a & b) + '*'; char d = (a | b) - '-'; char e = (a ^ b) + '+'; printf("%c %c %c \n", c, d, e); return 0; }
ASCII encoding for relevant characters is given below
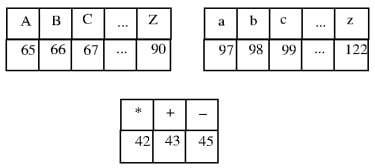
- A) z K S
- B) 122 75 83
- C) * – +
- D) P x +
Q11: What is printed by the following ANSI C program?
#include <stdio.h> int main(int argc, char *argv[]) { int a[3][3][3] = { {1, 2, 3, 4, 5, 6, 7, 8, 9}, {10, 11, 12, 13, 14, 15, 16, 17, 18}, {19, 20, 21, 22, 23, 24, 25, 26, 27} }; int i = 0, j = 0, k = 0; for(i = 0; i < 3; i++) { for(k = 0; k < 3; k++) { printf("%d ", a[i][j][k]); } printf(" \n"); } return 0; }
- A) 1 2 3
10 11 12
19 20 21 - B) 1 4 7
10 13 16
19 22 25 - C) 1 2 3
4 5 6
7 8 9 - D) 1 2 3
13 14 15
25 26 27
Q12): What is printed by the following ANSI C program?
#include <stdio.h> int main(int argc, char *argv[]) { int x = 1, z[2] = {10, 11}; int *p = NULL; p = &x; *p = 10; p = &z[1]; *(&z[0] + 1) += 3; printf("%d, %d, %d \n", x, z[0], z[1]); return 0; }
- A) 1, 10, 11
- B) 1, 10, 14
- C) 10, 14, 11
- D) 10, 10, 14
GATE CSE 2021 SET-2
Q13): Consider the following ANSI C program
#include <stdio.h> int foo(int x, int y, int q) { if ((x <= 0) && (y <= 0)) return q; if (x <= 0) return foo(x, y - q, q); if (y <= 0) return foo(x - q, y, q); return foo(x, y - q, q) + foo(x - q, y, q); } int main() { int r = foo(15, 15, 10); printf("%d", r); return 0; }
The output of the program upon execution is ____
Q14): Consider the following ANSI C code segment:
z=x + 3 + y-> f1 + y-> f2; for (i = 0; i < 200; i = i + 2) { if (z > i) { p = p + x + 3; q = q + y-> f1; } else { p = p + y-> f2; q = q + x + 3; } }
Assume that the variable y points to a struct (allocated on the heap) containing two fields f1 and f2, and the local variables x, y, z, p, q, and i are allotted registers. Common sub-expression elimination (CSE) optimization is applied on the code. The number of addition and the dereference operations (of the form y -> f1 or y -> f2) in the optimized code, respectively, are:
- A) 403 and 102
- B) 203 and 2
- C) 303 and 102
- D) 303 and 2
Q15): Consider the following ANSI C function:
int SomeFunction (int x, int y) { if ((x==1) || (y==1)) return 1; if (x==y) return x; if (x > y) return SomeFunction(x-y, y); if (y > x) return SomeFunction (x, y-x); }
The value returned by SomeFunction(15, 255) is __
Q16): Consider the following ANSI C program.
#include < stdio.h > int main( ) { int arr[4][5]; int i, j; for (i=0; i < 4; i++) { for (j=0; j < 5; j++) { arr[i][j] = 10 * i + j; } } printf("%d", *(arr[1]+9)); return 0; }
What is the output of the above program?
- A) 14
- B) 20
- C) 24
- D) 30
Also Read: Java Aptitude MCQ Questions with Answers for Freshers
More Practice: Data Structures Gate Questions and Answers PDF